Développement
Comment interagir avec Spot SDK
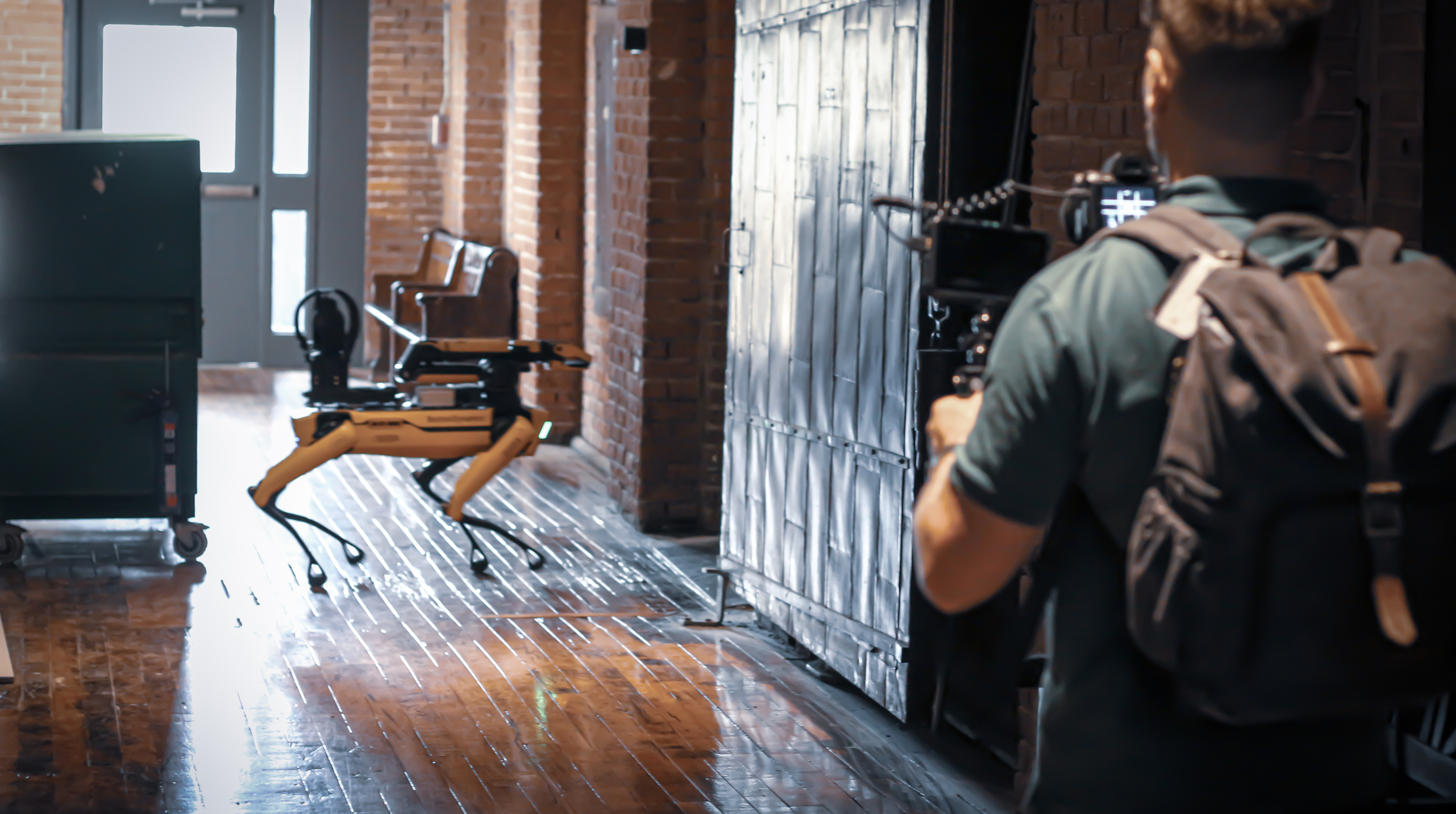
Comme vous le savez sans doute, nous sommes les fiers propriétaires d'un robot Spot. Ce fut un plaisir d'explorer cette nouvelle technologie et de découvrir comment elle peut combler les besoins de nos clients. Au fur et à mesure que nous en apprenons davantage sur Spot, nous voulons partager avec vous nos découvertes, et tout particulièrement la façon dont vous pouvez commander à Spot d’effectuer des tâches dans le contrôleur. Nous pouvons facilement y entrer avec l'aide de Spot SDK (software development kit) et du Knowledge Center fournis par Boston Dynamics.
Communiquer avec Spot
Premièrement, nous devons établir une connexion sécurisée avec Spot afin de le commander. Spot propose différentes méthodes, pour de meilleurs résultats, nous vous recommandons de vous connecter à son réseau Wifi 2,4 GHz. Notez que si vous faites la même chose, il serait idéal d'avoir plusieurs connexions Wifi (ou LAN) sur votre machine, car vous devrez probablement maintenir une connexion à votre Internet pour télécharger des ressources ou demander de l'aide en cours de route.
Une fois authentifié, nous pouvons maintenant envoyer un ping à son adresse IP avec succès.
Pour commander Spot, vous aurez également besoin d'un compte utilisateur et d'un mot de passe fonctionnels. Nous utiliserons des espaces réservés, super sécurisés, pour cet article.
Configuration de l'environnement
Techniquement, vous pouvez exécuter notre code python directement sur votre machine. Cependant, chez Osedea, nous aimons utiliser Docker, avec l'aide de Visual Studio Code Remote - Containers, c'est un rêve !
Tout d'abord, notre Dockerfile
doit contenir les éléments suivants:
Et notre docker-requirements.txt
contiendra les bibliothèques dont nous avons besoin pour communiquer avec Spot :
Maintenant, à partir de VSCode « Open Folder in Container » et laissez la magie opérer. Une fois le conteneur créé, vous pouvez confirmer que vous pouvez toujours vous connecter à Spot en envoyant une fois de plus un ping à son adresse IP :
Commander Spot
Maintenant que nous avons établi une connexion avec Spot et mis en place un environnement de travail, nous pouvons commencer !
Notez que l'extrait ci-dessus a été extrait du SDK mentionné ci-haut et supprimé. Nous vous invitons à jeter un œil à un exemple plus fonctionnel de hello spot.py.
Une fois cela fait, l'exécution de la commande suivante active Spot et le fait se tenir debout, poser, se tenir plus grand, puis s'éteindre [1].
Félicitations 🎉 ! Vous avez en théorie commandé avec succès votre Spot… cependant, si vous n'en avez pas, envoyez-nous un message et peut-être pourrez-vous rencontrer le nôtre.
[1] Pour ce faire vous devez techniquement établir une estop externe. Cela peut facilement se faire en suivant l’exemple suivant : Boston-Dynamic/spot-sdk
Crédit Photo: yakari_pixel

Cet article vous a donné des idées ? Nous serions ravis de travailler avec vous ! Contactez-nous et découvrons ce que nous pouvons faire ensemble.